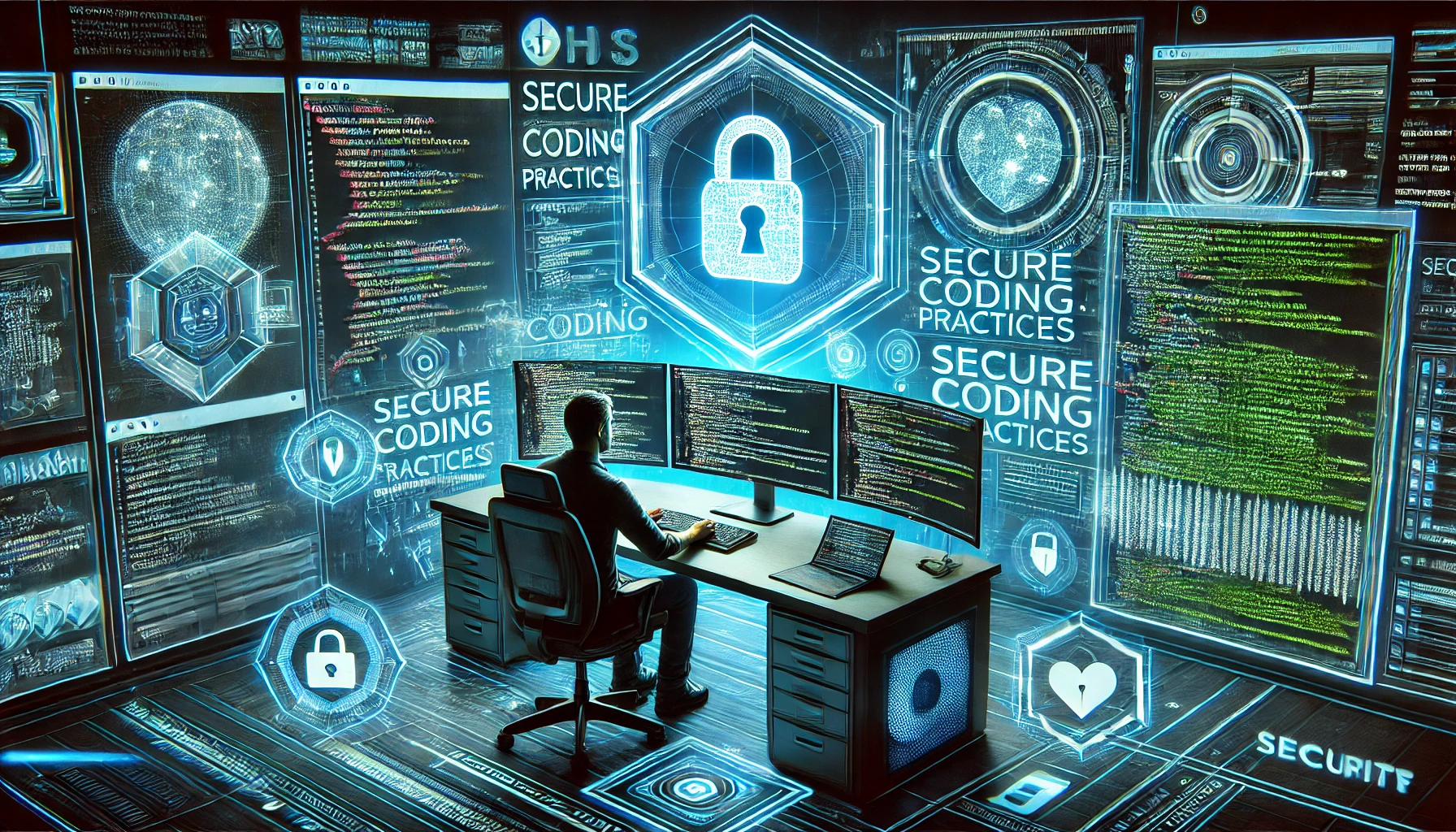
Introduction
Secure coding is essential to prevent security vulnerabilities that attackers can exploit. By following best practices, developers can build more resilient applications and reduce the risk of security breaches. This guide covers key secure coding principles and how to implement them effectively.
1. Input Validation
Risk: Unvalidated input can lead to injection attacks (SQLi, XSS, etc.)
Best Practices:
- Always validate and sanitize user input.
- Use whitelisting instead of blacklisting.
- Implement server-side validation along with client-side validation.
- Use built-in security libraries for input handling.
2. Least Privilege Principle
Risk: Excessive privileges can lead to unauthorized access and privilege escalation.
Best Practices:
- Grant only necessary permissions to users and applications.
- Use role-based access control (RBAC).
- Avoid using hardcoded credentials in source code.
- Implement multi-factor authentication (MFA) where applicable.
3. Secure Dependency Management
Risk: Using outdated or vulnerable libraries can introduce security flaws.
Best Practices:
- Regularly update dependencies to the latest secure versions.
- Use Software Composition Analysis (SCA) tools to detect vulnerabilities.
- Prefer digitally signed packages from trusted sources.
- Monitor CVE databases for reported security issues.
4. Secure Error Handling and Logging
Risk: Poor error handling can expose sensitive data or application logic.
Best Practices:
- Avoid exposing detailed error messages to users.
- Implement generic user-friendly error messages.
- Log errors securely with restricted access to logs.
- Use log monitoring tools to detect suspicious activities.
5. Secure Session Management
Risk: Insecure session handling can lead to session hijacking.
Best Practices:
- Use secure, HttpOnly, and SameSite cookies.
- Implement automatic session expiration.
- Regenerate session tokens after authentication.
- Use secure transport (HTTPS) for session communication.
6. Secure Configuration Management
Risk: Default configurations may expose unnecessary attack surfaces.
Best Practices:
- Disable unnecessary features and services.
- Enforce strong encryption settings.
- Store configuration secrets securely (use vault solutions).
- Implement automated security configuration scans.
7. Secure Coding Practices for APIs
Risk: Unprotected APIs can expose sensitive data.
Best Practices:
- Use OAuth 2.0 / OpenID Connect for authentication.
- Implement rate limiting and throttling to prevent abuse.
- Validate all API inputs and outputs.
- Use API gateways for enhanced security.
8. Avoid Hardcoded Secrets
Risk: Hardcoded credentials can be exposed in source control.
Best Practices:
- Store secrets in environment variables or secret management tools.
- Use Hashicorp Vault, AWS Secrets Manager, or Azure Key Vault.
- Implement automated secret scanning tools in CI/CD pipelines.
Conclusion
By following these secure coding practices, developers can significantly reduce vulnerabilities in their applications. Security should be integrated into every stage of the software development lifecycle (SDLC) to build robust and resilient applications.
Want to stay updated on the latest secure coding trends? Subscribe to our newsletter at Securebytesblog for expert insights!