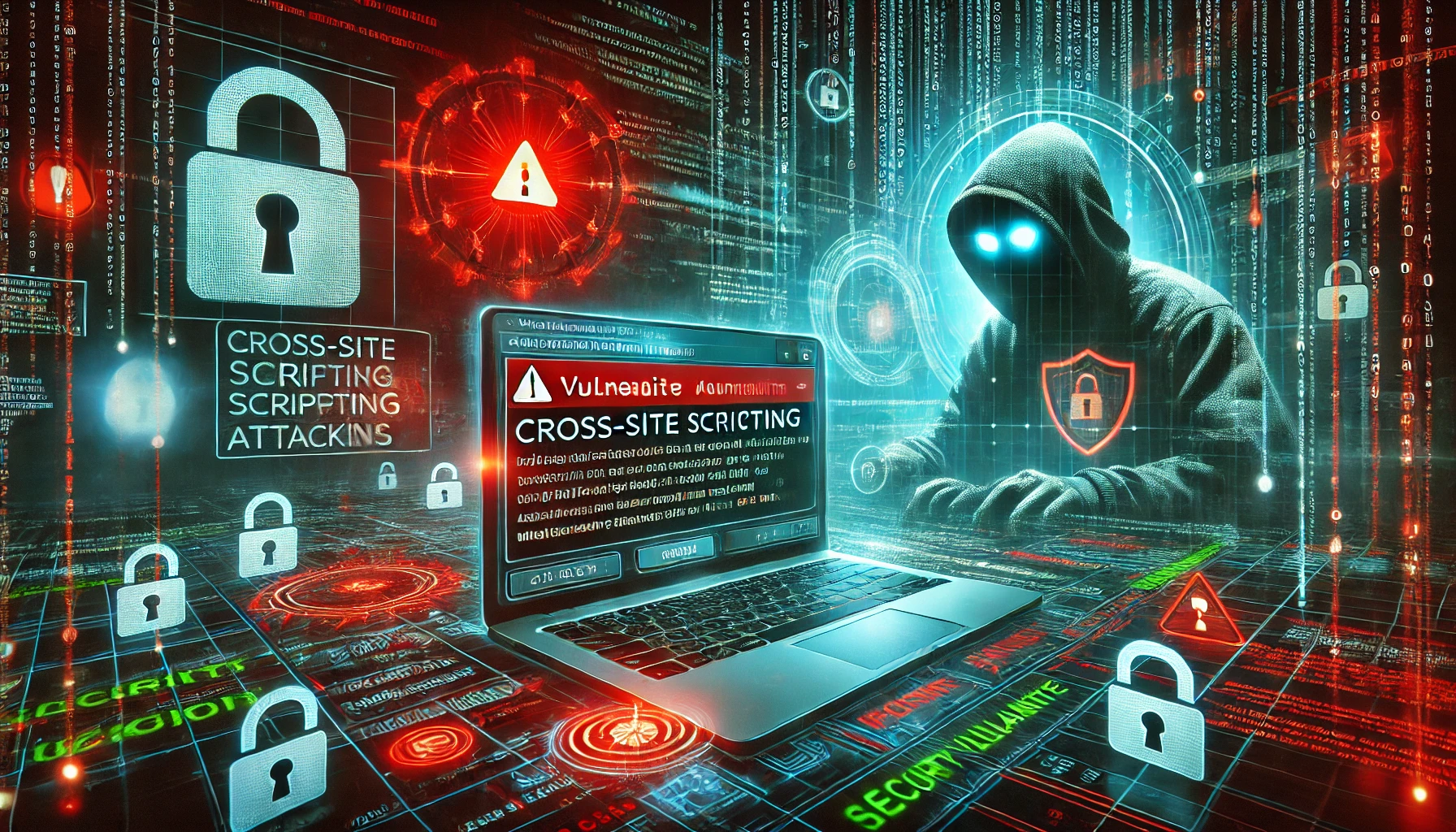
Introduction
Cross-Site Scripting (XSS) is one of the most common and dangerous web security vulnerabilities. It allows attackers to inject malicious scripts into web applications, potentially compromising user data, stealing session cookies, or defacing websites. This guide will explore how XSS attacks work, why your web application might be vulnerable, and how to effectively mitigate these risks.
1. What is Cross-Site Scripting (XSS)?
XSS occurs when an attacker injects malicious scripts into a website, which are then executed by unsuspecting users’ browsers. These scripts can steal sensitive information, redirect users to phishing sites, or perform actions on behalf of users without their consent.
Types of XSS Attacks
- Stored XSS: Malicious scripts are permanently stored on the server and served to users.
- Reflected XSS: Scripts are included in URL parameters and reflected back in the response.
- DOM-Based XSS: The vulnerability exists in the client-side JavaScript code that manipulates the Document Object Model (DOM).
2. How Attackers Exploit XSS Vulnerabilities
- Injecting scripts into comment fields, search bars, or user profile descriptions.
- Crafting malicious URLs containing JavaScript payloads and tricking users into clicking them.
- Manipulating insecure JavaScript functions that update the DOM.
- Exploiting poor input validation to execute arbitrary scripts.
3. How to Prevent XSS in Web Applications
3.1 Input Validation and Sanitization
- Validate and sanitize all user input before processing it.
- Use whitelisting (allow only expected characters) rather than blacklisting.
- Employ HTML escaping to neutralize special characters (
<
,>
,'
,"
,&
).
3.2 Secure Output Encoding
- Escape all dynamic content before rendering it in the browser.
- Use secure functions like
htmlspecialchars()
in PHP orencodeURIComponent()
in JavaScript. - Avoid injecting user-generated content directly into the DOM.
3.3 Implement Content Security Policy (CSP)
- Set a Content Security Policy (CSP) header to restrict the execution of unauthorized scripts.
- Example CSP policy:
Content-Security-Policy: default-src 'self'; script-src 'self' https://trustedsource.com
- Disallow inline scripts (
script-src 'self'
) to prevent execution of injected scripts.
3.4 Use Secure JavaScript Practices
- Avoid using
eval()
,document.write()
, orinnerHTML
with user input. - Prefer
textContent
overinnerHTML
to prevent script execution. - Implement secure event handlers (
addEventListener()
instead of inlineonclick
attributes).
3.5 Secure Cookies and Sessions
- Set
HttpOnly
andSecure
flags on session cookies to prevent theft. - Use
SameSite=Strict
to block CSRF attacks. - Regenerate session tokens after authentication.
3.6 Regular Security Audits and Testing
- Perform static analysis (SAST) using security tools like SonarQube or Snyk.
- Conduct dynamic analysis (DAST) using tools like OWASP ZAP or Burp Suite.
- Regularly update dependencies and frameworks to patch security vulnerabilities.
4. Detecting and Mitigating XSS Attacks in Real Time
- Implement Web Application Firewalls (WAFs) to filter malicious requests.
- Enable real-time logging and monitoring for suspicious activities.
- Use security headers like
X-XSS-Protection: 1; mode=block
(for legacy browsers).
Conclusion
Cross-Site Scripting (XSS) remains a prevalent security risk for web applications, but it can be effectively mitigated through input validation, output encoding, secure JavaScript practices, and Content Security Policies (CSP). By following these best practices, developers can build secure applications that protect users from malicious attacks.
Want to stay ahead of security threats? Subscribe to our newsletter at Securebytesblog for expert insights!